Introduction
Vue.js is a popular JavaScript framework for building user interfaces. It offers a balance between simplicity and power, making it a great choice for projects of all sizes. Create Vue App is perfect starting point! In this comprehensive guide, we’ll walk through everything you need to know to kickstart your Vue journey with npm, npx, yarn and Vue CLI setup.
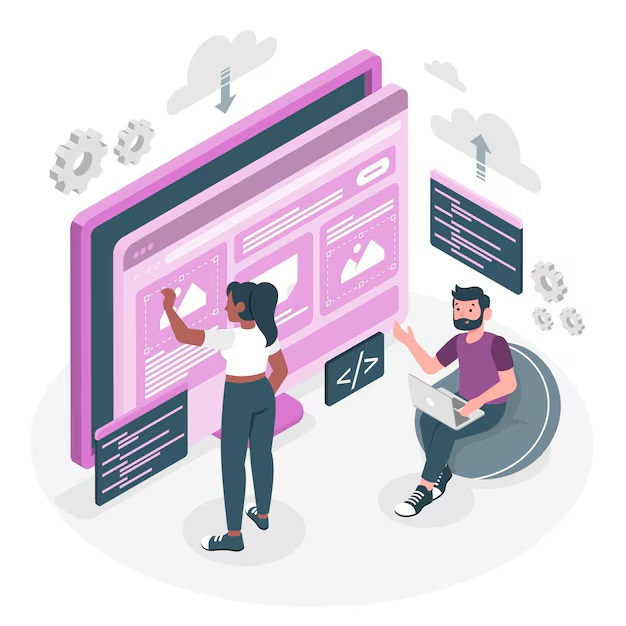
What is Vue CLI to Create Vue App?
Vue CLI is a command line tool which help Frontend developers quickly build a new Vue application. It provides a pre-configured environment with all necessary tools pre-installed, including Babel for modern JS features, Webpack for tie together your code, and ESLint for catching errors early on. With Vue CLI, you can focus on writing code and building features instead of focusing on configuration and basic code.
Getting Started the Create Vue App
You can create Vue app in three different ways:
Method #1: Using npm
- Ensure you have Node.js and npm installed on your system. If you don’t have, download them from the official Node.js website https://nodejs.org/en.
- Open your terminal or command prompt and navigate to the directory where you want to create the project. (Note: If you are not sure about Node.js, run command in terminal or command prompt –
node -v
) - Run the following command, replacing
my-app
with your designed project name and select select “Manually select features” and follow the steps as per requirement:
npm create vue my-app
Method #2: Using npx (Recommended for Beginners)
npx
comes bundled with npm versions 5.2 and above, so you don’t need to install any additional tools.
- Open your terminal and navigate to the directory where you want to create the project.
- Run the following command, replacing
my-app
with your designed project name
npx @vue/cli create my-app
Method #3: Using yarn
- Ensure you have Yarn as a package manager installed on your system. If you don’t have, download them from the official Yarn website https://classic.yarnpkg.com/lang/en/docs/install/
- Open your terminal or command prompt and navigate to the directory where you want to create the project.
- Run the following command, replacing
my-app
with your designed project name:
yarn create vue my-app
Method #4: Using npm and Vue CLI
- Ensure you have Node.js and npm installed on your system. If you don’t have, download them from the official Node.js website https://nodejs.org/en.
- Ensure you have Vue CLI installed on your system. If you don’t have, install by the command
npm install -g @vue/cli
- Open your terminal or command prompt and navigate to the directory where you want to create the project. (Note: If you are not sure about Node.js, run command in terminal or command prompt –
node -v
and If you are not sure about Node.js, run command in terminal or command prompt –vue --version
) - Run the following command, replacing
my-app
with your designed project name and select select “Manually select features” and follow the steps as per requirement:
vue create my-app
Exploring the Project Structure
Once your project is set up, take a moment to familiarize yourself with the project structure:
npm and Yarn
my-app/
├── node_modules/
├── public/
│ ├── index.html
│ └── favicon.ico
├── src/
│ ├── main.js
│ ├── App.vue
│ ├── components/
│ │ └── Header.vue
│ ├── assets/
│ │ └── logo.png
├── package.json
└── README.md
npx
my-app/
├── node_modules/
├── public/
│ ├── index.html
│ └── favicon.ico
├── src/
│ ├── main.js
│ ├── App.vue
│ ├── components/
│ │ └── Header.vue
│ ├── assets/
│ │ └── logo.png
└── README.md
public/
: This directory contains the HTML file and other assets like images, that are publicly accessible.src/
: All of your Vue components and JavaScript files will live here.package.json
: This file holds metadata about your project and dependencies.node_modules/
: This directory contains all the project’s dependencies.
Running Your Vue App
Now that your project is set up. Navigate to your project directory and run the following command:
npm run serve
(If you used Yarn, the command would be yarn serve
)
This command starts the development server, which typically runs on http://localhost:8080
by default.
Exploring the src
Folder: Building your app
The src
folder is where the real development happens. It has all the essential components that set up your Vue application. Let’s delve into some key elements within this folder:
Components:
Vue applications are constructed from reusable components. each component is a self contained unit responsible for rendering a specific part of the UI. You can create multiple components to represent different sections of your app like the header and login form.
For example, let’s create a Header.vue
component:
<template>
<header>
<h1>Welcome to My App</h1>
</header>
</template>
<script>
export default {
// Component logic goes here
}
</script>
<style scoped>
/* Component-specific styles go here */
header {
background-color: #333;
color: white;
padding: 20px;
}
h1 {
margin: 0;
}
</style>
App.vue:
This file serves as the entry point for your application. It typically renders the main component that defines the overall structure of your app.
<template>
<div>
<Header />
<!-- Other components -->
</div>
</template>
<script>
import Header from './components/Header.vue';
export default {
components: {
Header
// Additional components go here
}
}
</script>
<style scoped>
/* Global styles go here */
</style>
Useful Dependencies to Create Vue App:
When setting up a Vue app, you’ll need to install essential dependencies to build and run your application effectively.
- Vue: The core library for Vue applications.
- Vue Router: Provides navigation capabilities for single-page applications.
- Vuex: A state management pattern and library for Vue.js applications.
- Babel: Facilitates the utilization of modern JavaScript features and JSX syntax.
- Webpack: A module bundler for compiling and bundling JavaScript files, CSS, and other assets.
- ESLint: A static code analysis tool for identifying and reporting patterns in JavaScript code.
If using npm (Node Package Manager):
npm install vue vue-router vuex @babel/core @babel/preset-env webpack webpack-cli eslint --save-dev
If using yarn:
yarn add vue vue-router vuex @babel/core @babel/preset-env webpack webpack-cli eslint --dev
Conclusion
Congratulations! You’ve successfully set up your first Vue project using either npm, npx, yarn and Vue CLI. From here, the sky’s the limit. Experiment with different components, explore third-party libraries, and start building amazing web applications. Happy coding!
Remember: The Vue ecosystem is vast and ever-evolving. Stay curious, explore the official Vue documentation https://vuejs.org/guide/introduction.html , experiment with different libraries and tools, and actively engage with the vibrant Vue community. With dedication and practice, you’ll be building remarkable Vue applications in no time!
Stay tuned every Tuesday for fresh insights into frontend topics, because keeping up with the latest in web development has never been more exciting!