Java’s Stream API has been a game-changer for developers, letting us work with data in a more concise and functional way. But within the Streams realm lies a hidden gem: the Key Stream API methods. Buckle up, Java enthusiasts, because we’re about to unleash its potential in 2024!
Stream API: Your Coding Superpower
Imagine you’re stuck at a party where everyone keeps telling the same joke. That’s what traditional Java code can feel like sometimes—repetitive and tedious. The Key Stream API swoops in like a knight in shining armor, offering a way to avoid redundancies and write cleaner, more expressive code. Basically a stream in Java can be defined as a sequence of elements from a source (collections) that supports aggregate operations like filtering, finding max, min, sum, etc.
The idea is that a user will extract only the values they require from a stream, and these elements are only produced invisibly to the user as and when required. This is a form of producer-consumer relationship.
A stream pipeline consists of a source, followed by zero or more intermediate operations, and a terminal
operation, The java.util.stream package is introduced in Java 8, which contains classes and interfaces to support such functional-style operations on streams of elements.
- Streams can be created from collections, Lists, Sets, arrays, lines of a file etc.
- Intermediate operations such as filter, map and sorted return a new stream so that we can chain multiple operations.
- Terminal operations such as forEach, collect, and reduce are either void or return a non-stream result.
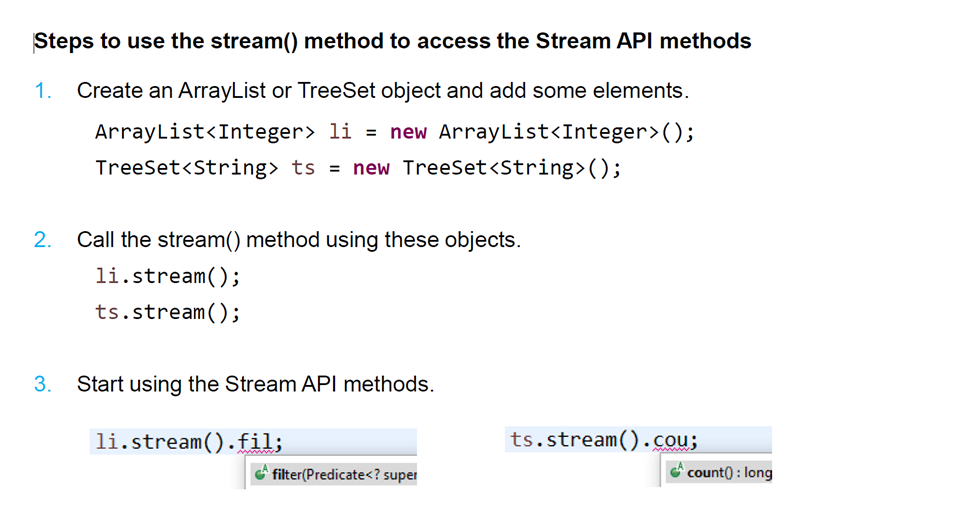
Fluent and Fabulous: Key Stream API methods to Master
Now, let’s talk about some of the coolest key stream API methods:
filter()
This little method lets you sift through your data and pick out only the elements that meet certain criteria. It’s like having a virtual sieve for your data—only the good stuff gets through. A predicate (condition) is called for each element in the stream.
collect()
Once you’ve worked your magic on your data, it’s time to gather it all up and put it back together again. That’s where the collect() method comes in handy. It allows you to collect your data into a variety of different data structures, so you can organize it however you like. Functions from the Collectors class are passed as arguments to mention how the elements have to be packed. For example, Collectors.toList() will accumulate the elements into an ArrayList.
// filter and collect example
List<String> heroes = Arrays.asList("Superman", "Batman", "Wonder Woman", "Aquaman");
List<String> superHeroes = heroes.stream()
.filter(hero -> hero.startsWith("Super"))
.collect(Collectors.toList());
System.out.println(superHeroes); // Output: [Superman]
forEach()
forEach() was introduced in Java 8 to iterate a collection of elements that is defined in the iterable interface. The signature of forEach in the iterable interface is default void forEach(Consumer<super T> action), and we can pass a lambda expression as an argument.
List<String> animals = Arrays.asList("Dog", "Cat", "Bird", "Fish");
// Using forEach to print each animal in the list
animals.forEach(animal -> System.out.println("I am a " + animal));
reduce()
Feeling overwhelmed by all that data? Don’t worry, the reduce() method is here to save the day! This handy little tool lets you condense your data into a single value, making it easier to manage and understand. It’s like packing a suitcase for a trip; you want to fit everything you need into one neat package. With the reduce method, you can do just that!
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println("Sum of numbers: " + sum); // Output: Sum of numbers: 15
count()
Returns the count of elements in the given stream that satisfy the given filter condition. Examples of scenarios where this method will be useful are when a count of how many students have cleared the test is required or when a count of how many employees are working onsite is required.
// Create a list of integers
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
numbers.add(4);
numbers.add(5);
// Count the number of elements in the list
long count = numbers.stream().count();
// Print the count
System.out.println("Number of elements in the list: " + count);
max() and min()
Returns the maximum or minimum element of the given stream according to the provided comparator. It returns an Optional instance describing the maximum element or minimum element of this stream, or an empty Optional if the stream is empty. Use the optional class get() method to fetch the actual value.
// Example using an array
Integer[] numbersArray = {1, 5, 3, 7, 2};
Optional<Integer> maxFromArray = Arrays.stream(numbersArray).max(Integer::compareTo);
Optional<Integer> minFromArray = Arrays.stream(numbersArray).min(Integer::compareTo);
System.out.println("Max value from array: " + maxFromArray.orElse(null));
System.out.println("Min value from array: " + minFromArray.orElse(null));
// Example using a list
List<Integer> numbersList = Arrays.asList(1, 5, 3, 7, 2);
Optional<Integer> maxFromList = numbersList.stream().max(Integer::compareTo);
Optional<Integer> minFromList = numbersList.stream().min(Integer::compareTo);
System.out.println("Max value from list: " + maxFromList.orElse(null));
System.out.println("Min value from list: " + minFromList.orElse(null));
map()
Ready to transform your data into something truly spectacular? Meet the map() method! With just a few lines of code, you can morph your data into whatever shape you desire. The intermediate operation map converts each element in the stream into another object via the given function. It returns a new stream consisting of the results of applying the given function to the elements of the given stream.
// Example using a list of strings
List<String> names = Arrays.asList("John", "Alice", "Bob", "Emily");
// Transform each name to uppercase using map() method
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase) // Function to transform each string to uppercase
.collect(Collectors.toList());
// Print the transformed names
System.out.println("Original names: " + names);
System.out.println("Names in uppercase: " + upperCaseNames);
limit(), skip() and sorted()
The limit() method is used to limit the number of elements in a stream to a specified maximum size. It ensures that only the first n elements are processed in the stream. The skip() method is used to skip a specified number of elements in a stream. It ignores the first n elements and starts processing from the (n+1)th element onward. The sorted()
method is used to sort the elements of a stream based on a specified comparator or using natural ordering if no comparator is provided.
// Example array of integers
int[] numbers = {5, 2, 8, 1, 9, 4, 3, 7, 6};
// Using limit(): Get the first 5 elements of the array
System.out.println("Using limit(): Get the first 5 elements of the array");
Arrays.stream(numbers)
.limit(5)
.forEach(System.out::println);
// Using skip(): Skip the first 3 elements of the array
System.out.println("Using skip(): Skip the first 3 elements of the array");
Arrays.stream(numbers)
.skip(3)
.forEach(System.out::println);
// Using sorted(): Sort the elements of the array
System.out.println("Using sorted(): Sort the elements of the array");
Arrays.stream(numbers)
.sorted() // natural order
.forEach(System.out::println);
conclusion
With these key stream API methods in your toolkit, there’s no limit to what you can accomplish as a Java developer. So go forth, brave coder, and unlock the full potential of Java with filter(), map(), and reduce(). Your coding adventures await!
Remember, with great power comes great responsibility and a whole lot of fun! Happy coding, Java heroes! 🚀✨. For more Java insights and tutorials, check out this link.