In this step-by-step guide, you’ll learn how to upload Excel files to your Spring Boot application using Postman. Uploading Excel files to a Spring Boot application is a common requirement for many projects that involve data import and export functionalities. I assume you have a basic understanding of Spring boot and Postman. I’ll cover the backend setup and the Postman configuration to make this process simple.
Setting Up Your Spring Boot Project
First, you have to create a new Spring Boot project. You can use Spring Initializr to generate your project. Include the following dependencies.
- Spring Web
- Spring Boot DevTools
- Apache POI (for handling Excel files)
Once your project is generated and imported into your IDE (Spring tool suite or Intellij), you’ll need to add the Apache POI dependency to your pom.xml if it’s not already included:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.0.0</version>
</dependency>
Creating the Controller
Next, create a REST controller to handle the file upload. Create a new class named FileUploadController.java in your controller package.
package com.example.demo.controller;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return new ResponseEntity<>("Please select a file to upload.", HttpStatus.BAD_REQUEST);
}
try {
Workbook workbook = new XSSFWorkbook(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
Iterator<Row> rows = sheet.iterator();
List<String> data = new ArrayList<>();
while (rows.hasNext()) {
Row currentRow = rows.next();
Iterator<Cell> cellsInRow = currentRow.iterator();
while (cellsInRow.hasNext()) {
Cell currentCell = cellsInRow.next();
data.add(currentCell.toString());
}
}
workbook.close();
data.forEach(System.out::println);
// Do something with the data (e.g., save to database, or perform some validation)
return new ResponseEntity<>("File uploaded successfully!", HttpStatus.OK);
} catch (IOException e) {
return new ResponseEntity<>("An error occurred while processing the file.", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
The controller listens for POST requests at /api/upload. It checks if a file is provided, processes the Excel file using Apache POI, and returns a success message if everything goes well.
Configuring Postman
Using Postman to test the Excel file upload functionality in your Spring Boot application ensures that your implementation works smoothly and can handle real-world data scenarios. Now that your backend is set up, you can use Postman to test the file upload
- Open Postman and create a new
POST
request. - Enter the URL:
http://localhost:8080/api/upload
- Select the Body tab and choose
form-data
. - Add a key named
file
, set the type toFile
, and upload an Excel file from your computer. - Send the request.
If everything is configured correctly, you should receive a response indicating the file was uploaded successfully, something like this below
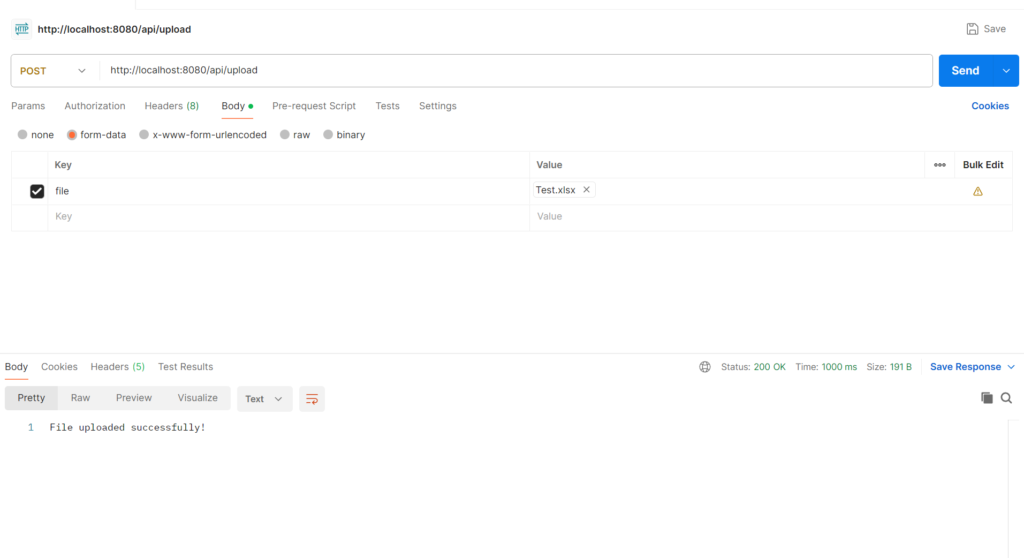
Testing and Verifying
To ensure everything is working:
- Start your Spring Boot application.
- Send the POST request from Postman with an Excel file.
- Check the response: You should see a success message.
You can also add some logging in your controller to print the data read from the Excel file to the console. This will help verify that the file is being read correctly. Congratulations! You just learned to upload excel to Spring Boot using Postman.
Conclusion
Uploading and importing an Excel file into a Spring Boot application using Postman is a simple process. By following the steps above, you can quickly set up a file upload endpoint and test it with Postman. You can efficiently handle Excel file uploads in your Spring Boot applications, making your data management tasks simpler and more robust. You can also customize the setup to include more complex processing, like saving the data into the database after performing some validation.