Introduction
Welcome to the world of Vue.js, where every click, hover, and keystroke brings your web applications to life! In this blog post, we’re diving deep into the heartbeat of Vue.js: its events [Part 2] (Visit Vue.js Event [Part 1] ). Imagine being able to respond instantly to a user’s action, whether it’s clicking a button, typing in a form, or scrolling through a page. With Vue.js events, that’s exactly what you can do. Get ready to discover essential Vue.js events, each with its own superpower to make your applications more interactive, engaging, and downright irresistible. Let’s unlock the magic of Vue.js events together!
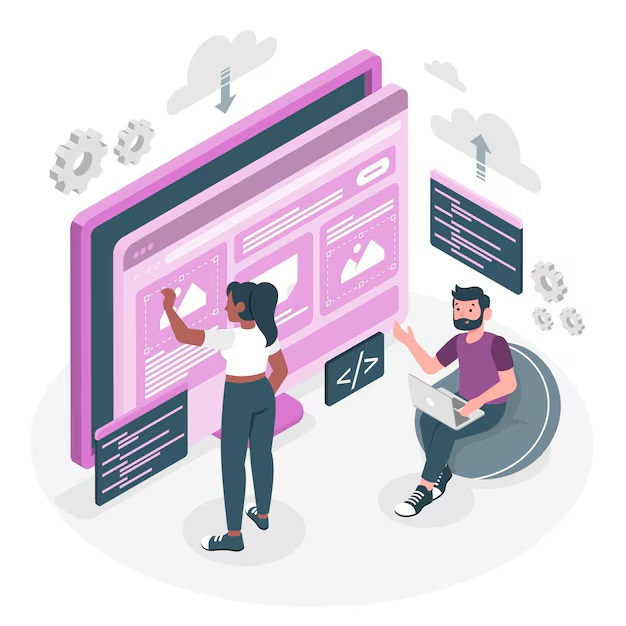
Vue.js Events
Up and Down Keystroke Handling Vue.js Events
@keydown
- Use: Triggers when a key is pressed down, essential for implementing keyboard shortcuts, navigation, and form interactions.
- Example:
<template>
<input type="text" @keydown="handleKeyDown">
</template>
<script>
export default {
methods: {
handleKeyDown(event) {
console.log('Key pressed:', event.key);
}
}
}
</script>
- Issue: Handling keydown events without proper consideration for keyboard accessibility may exclude users with disabilities.
- Solution: Follow accessibility best practices and ensure keyboard navigation alternatives for mouse-driven interactions.
@keyup
- Use: Triggers when a key is released, useful for implementing keyboard shortcuts, form validation, and interactive elements.
- Example:
<template>
<input type="text" @keyup="handleKeyUp">
</template>
<script>
export default {
methods: {
handleKeyUp(event) {
console.log('Key released:', event.key);
}
}
}
</script>
- Issue: Mishandling keyup events may result in unexpected behavior or inconsistent user experiences, particularly in complex interfaces.
- Solution: Maintain consistent keyup event handling and provide clear guidance on supported keyboard interactions.
Up Keystroke Handling Vue.js Events
@keyup.escape
- Use: Fires when the Escape key is released, commonly used for closing modals, canceling actions, and dismissing overlays.
- Example:
<template>
<input type="text" @keyup.escape="handleEscape">
</template>
<script>
export default {
methods: {
handleEscape() {
console.log('Escape key released!');
}
}
}
</script>
- Issue: Inconsistent handling of Escape key events may confuse users or lead to unexpected behaviors, especially in modal dialogs.
- Solution: Implement clear and consistent Escape key handling to ensure intuitive user interactions and accessibility.
Press and Released Keystroke Handling Vue.js Events
@keypress
- Use: Fires when a key is pressed down and immediately released, commonly used for handling text input and form validation.
- Example:
<template>
<input type="text" @keypress="handleKeyPress">
</template>
<script>
export default {
methods: {
handleKeyPress(event) {
console.log('Key pressed and released:', event.key);
}
}
}
</script>
- Issue: Inconsistent handling of keypress events across different browsers and devices may lead to usability issues or data discrepancies.
- Solution: Test keypress event handling across browsers and devices, and implement fallbacks or polyfills as needed for consistent behavior.
Key with Down Keystroke Handling Vue.js Events
@keydown.enter
- Use: Executes when the Enter key is pressed, commonly used for form submission, search functionality, and modal interactions.
- Example:
<template>
<input type="text" @keydown.enter="handleSubmit">
</template>
<script>
export default {
methods: {
handleSubmit() {
console.log('Enter key pressed!');
}
}
}
</script>
- Issue: Mishandling Enter key events may lead to unintentional form submissions or inconsistent user experiences.
- Solution: Implement explicit handling for Enter key events, considering context and user expectations.
@keydown.tab
- Use: Triggers when the Tab key is pressed, useful for managing focus order, navigation, and accessibility.
- Example:
<template>
<input type="text" @keydown.tab="handleTab">
</template>
<script>
export default {
methods: {
handleTab() {
console.log('Tab key pressed!');
}
}
}
</script>
- Issue: Mishandling Tab key events may disrupt keyboard navigation or cause focus trapping in complex interfaces.
- Solution: Ensure consistent and predictable focus behavior by handling Tab key events appropriately.
@keydown.space
- Use: Triggers when the Space key is pressed, commonly used for activating buttons, toggling checkboxes, and playing/pausing media.
- Example:
<template>
<button @keydown.space="handleSpace">Press space</button>
</template>
<script>
export default {
methods: {
handleSpace() {
console.log('Space key pressed!');
}
}
}
</script>
- Issue: Mishandling Space key events may result in inconsistent behavior or conflicts with other interactions.
- Solution: Ensure proper event handling and consider user expectations for consistent interactions.
Arrow Key with Down Keystroke Handling Vue.js Events
@keydown.arrowup
- Use: Executes when the Arrow Up key is pressed, useful for implementing keyboard navigation, slider controls, and menu selection.
- Example:
<template>
<div @keydown.arrowup="handleArrowUp">Press arrow up</div>
</template>
<script>
export default {
methods: {
handleArrowUp() {
console.log('Arrow Up key pressed!');
}
}
}
</script>
- Issue: Mishandling Arrow Up key events may lead to unexpected behavior or conflicts with other keyboard shortcuts.
- Solution: Implement arrow key event handling judiciously and consider user expectations for consistent interactions.
@keydown.arrowdown
- Use: Triggers when the Arrow Down key is pressed, useful for implementing keyboard navigation, slider controls, and menu selection.
- Example:
<template>
<div @keydown.arrowdown="handleArrowDown">Press arrow down</div>
</template>
<script>
export default {
methods: {
handleArrowDown() {
console.log('Arrow Down key pressed!');
}
}
}
</script>
- Issue: Mishandling Arrow Down key events may result in unexpected behavior or conflicts with other keyboard shortcuts.
- Solution: Ensure proper event handling and consider user expectations for consistent interactions.
Click Handling Vue.js Events
@click.right
- Use: Triggers when the right mouse button is clicked, often used as an alternative to contextmenu for opening custom context menus.
- Example:
<template>
<div @click.right.prevent="handleRightClick">Right-click me</div>
</template>
<script>
export default {
methods: {
handleRightClick(event) {
console.log('Right-clicked!');
// Implement custom context menu logic
}
}
}
</script>
- Issue: Incorrect handling of right-click events may lead to unexpected behavior or interfere with default browser context menus.
- Solution: Ensure consistent behavior across browsers and provide appropriate fallbacks for non-standard mouse inputs.
Context Menu Handling Vue.js Events
@contextmenu
- Use: Executes when the right mouse button is clicked to open the context menu, commonly used for custom context menus and interactive elements.
- Example:
<template>
<div @contextmenu="handleContextMenu">Right-click here</div>
</template>
<script>
export default {
methods: {
handleContextMenu(event) {
event.preventDefault(); // Prevent default context menu
console.log('Context menu opened!');
}
}
}
</script>
- Issue: Mishandling contextmenu events may interfere with default browser behavior or cause confusion for users.
- Solution: Implement contextmenu event handling carefully and ensure clear visual cues for custom context menus.
@contextmenu.prevent
- Use: Executes when the right mouse button is clicked to open the context menu, commonly used for custom context menus and interactive elements.
- Example:
<template>
<div @contextmenu="handleContextMenu">Right-click here</div>
</template>
<script>
export default {
methods: {
handleContextMenu(event) {
event.preventDefault(); // Prevent default context menu
console.log('Context menu opened!');
}
}
}
</script>
- Issue: Mishandling contextmenu events may interfere with default browser behavior or cause confusion for users.
- Solution: Implement contextmenu event handling carefully and ensure clear visual cues for custom context menus.
Conclusion
The power of interactivity is in your hands. Armed with the knowledge of essential events, you’re ready to captivate users with seamless experiences that respond to their every move. Whether it’s handling clicks, tracking mouse movements, or managing keyboard shortcuts, Vue.js events empower you to create applications that feel intuitive, responsive, and downright delightful. So go ahead, experiment, explore, and let the magic of Vue.js events elevate your next project to new heights. Explore Vue.js 2 and Vue.js 3 .
Remember: The Vue ecosystem is vast and ever-evolving. Stay curious, explore the official Vue documentation https://vuejs.org/guide/introduction.html , experiment with different libraries and tools, and actively engage with the vibrant Vue community. With dedication and practice, you’ll be building remarkable Vue applications in no time!
Stay tuned every Tuesday for fresh insights into frontend topics, because keeping up with the latest in web development has never been more exciting!