Introduction
Welcome to the world of Vue.js, where every click, hover, and keystroke brings your web applications to life! In this blog post, we’re diving deep into the heartbeat of Vue.js: its events [Part 1] (Visit Vue.js Events [Part 2] ). Imagine being able to respond instantly to a user’s action, whether it’s clicking a button, typing in a form, or scrolling through a page. With Vue.js events, that’s exactly what you can do. Get ready to discover essential Vue.js events, each with its own superpower to make your applications more interactive, engaging, and downright irresistible. Let’s unlock the magic of Vue.js events together!
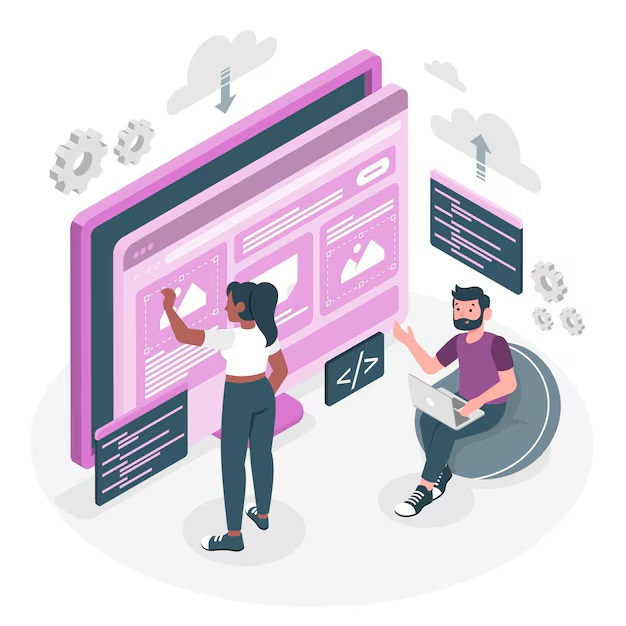
Vue.js Events
Most Used Handling Vue.js Events: Every Vue.js developer need to know
@click
- Use: Triggers a method when an element is clicked, commonly used for buttons, links, and interactive elements.
- Example:
<template>
<button @click="handleClick">Click me</button>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('Button clicked!');
}
}
}
</script>
- Issue: Overuse of click events may lead to an overly responsive interface, causing accidental triggers or user frustration.
- Solution: Use click events sparingly and provide visual feedback to confirm user actions.
@input
- Use: Fires when the value of an input changes, useful for real-time validation and updating data bindings.
- Example:
<template>
<input type="text" @input="handleInput">
</template>
<script>
export default {
methods: {
handleInput(event) {
console.log(event.target.value);
}
}
}
</script>
- Issue: Continuous firing of input events during typing may impact performance, especially with complex validation logic.
- Solution: Debounce input events or use libraries like
lodash.debounce
to optimize performance.
@submit
- Use: Executes when a form is submitted, commonly used for form validation, data submission, and navigation.
- Example:
<template>
<form @submit.prevent="handleSubmit">
<button type="submit">Submit</button>
</form>
</template>
<script>
export default {
methods: {
handleSubmit() {
console.log('Form submitted!');
}
}
}
</script>
- Issue: Handling form submission events without proper validation may lead to security vulnerabilities or data inconsistency.
- Solution: Implement client-side and server-side validation to ensure data integrity and protect against malicious inputs.
@change
- Use: Fires when the value of an input changes and the element loses focus, commonly used for select dropdowns, checkboxes, and radio buttons.
- Example:
<template>
<input type="text" @change="handleChange">
</template>
<script>
export default {
methods: {
handleChange(event) {
console.log('Input changed to:', event.target.value);
}
}
}
</script>
- Issue: Inconsistencies in handling change events across different input types may lead to usability issues or data discrepancies.
- Solution: Maintain consistent behavior for change events and provide clear feedback to users when values change.
Effect Handling Vue.js Events
@scroll
- Use: Fires when the element is scrolled, useful for lazy loading content, infinite scrolling, and parallax effects.
- Example:
<template>
<div style="height: 200px; overflow-y: scroll" @scroll="handleScroll">
Scroll me
</div>
</template>
<script>
export default {
methods: {
handleScroll() {
console.log('Scrolled!');
}
}
}
</script>
- Issue: Excessive use of scroll events may degrade performance, especially on mobile devices or with large datasets.
- Solution: Optimize scroll event listeners and consider alternatives like Intersection Observer for performance-critical scenarios.
@focus
- Use: Triggers when an element gains focus, commonly used for form fields, modals, and interactive elements.
- Example:
<template>
<input type="text" @focus="handleFocus">
</template>
<script>
export default {
methods: {
handleFocus() {
console.log('Input focused!');
}
}
}
</script>
- Issue: Overuse of focus events may disrupt the natural flow of user interaction, causing unexpected behavior.
- Solution: Use focus events judiciously and provide clear visual cues to indicate focus changes.
@blur
- Use: Executes when an element loses focus, useful for form validation, input masking, and interactive elements.
- Example:
<template>
<input type="text" @blur="handleBlur">
</template>
<script>
export default {
methods: {
handleBlur() {
console.log('Input blurred!');
}
}
}
</script>
- Issue: Mishandling blur events may lead to accessibility issues, particularly for users navigating with screen readers or keyboard-only controls.
- Solution: Ensure proper focus management and provide alternative ways to interact with blurred elements.
Most Used Mouse Handling Vue.js Events
@mouseleave
- Use: Executes when the mouse cursor leaves an element, useful for hover effects, tooltips, and interactive elements.
- Example:
<template>
<div @mouseleave="handleMouseLeave">Mouse leave here</div>
</template>
<script>
export default {
methods: {
handleMouseLeave() {
console.log('Mouse left!');
}
}
}
</script>
- Issue: Improper use of mouseleave events may cause accessibility issues for users navigating with assistive technologies.
- Solution: Ensure consistent behavior for mouseleave events and test with accessibility tools to verify compatibility.
@mouseover
- Use: Triggers when the mouse cursor moves over an element, commonly used for tooltips, hover effects, and interactive elements.
- Example:
<template>
<div @mouseover="handleMouseOver">Hover over me</div>
</template>
<script>
export default {
methods: {
handleMouseOver() {
console.log('Mouse over me!');
}
}
}
</script>
- Issue: Excessive use of mouseover events may clutter the user interface or lead to unintended interactions, especially on touch devices.
- Solution: Use mouseover events judiciously and provide clear visual cues to guide user interactions.
@mouseout
- Use: Executes when the mouse cursor leaves an element, useful for hover effects, tooltips, and interactive elements.
- Example:
<template>
<div @mouseout="handleMouseOut">Mouse out me</div>
</template>
<script>
export default {
methods: {
handleMouseOut() {
console.log('Mouse out me!');
}
}
}
</script>
- Issue: Mishandling mouseout events may cause unexpected behavior or disrupt user interactions, particularly in complex interfaces.
- Solution: Implement consistent mouseout event handling and test thoroughly to ensure desired behavior across devices.
@mouseenter
- Use: Triggers when the mouse cursor enters an element, commonly used for hover effects, tooltips, and interactive elements.
- Example:
<template>
<div @mouseenter="handleMouseEnter">Mouse enter here</div>
</template>
<script>
export default {
methods: {
handleMouseEnter() {
console.log('Mouse entered!');
}
}
}
</script>
- Issue: Mishandling mouseenter events on nested elements can lead to unexpected behavior, such as multiple firing of event handlers.
- Solution: Ensure proper event propagation and consider event delegation for complex DOM structures.
Other Mouse Handling Vue.js Events
@mousedown
- Use: Triggers when a mouse button is pressed down over an element, useful for implementing drag-and-drop functionality, custom context menus, and interactive elements.
- Example:
<template>
<div @mousedown="handleMouseDown">Press and hold</div>
</template>
<script>
export default {
methods: {
handleMouseDown() {
console.log('Mouse button pressed down!');
}
}
}
</script>
- Issue: Excessive use of mousedown events without proper handling may interfere with default browser behavior or cause unintended side effects.
- Solution: Implement mousedown event handling judiciously and consider user expectations for consistent interactions.
@mouseup
- Use: Executes when a mouse button is released after being pressed down, commonly used for implementing interactive elements and triggering actions.
- Example:
<template>
<div @mouseup="handleMouseUp">Release mouse button</div>
</template>
<script>
export default {
methods: {
handleMouseUp() {
console.log('Mouse button released!');
}
}
}
</script>
- Issue: Mishandling mouseup events may lead to inconsistent user experiences or unexpected behavior, especially in complex interfaces.
- Solution: Ensure proper event handling and consider user expectations for consistent interactions across devices and browsers.
@mousemove
- Use: Triggers when the mouse cursor is moved over an element, useful for tracking mouse movement and implementing interactive features.
- Example:
<template>
<div @mousemove="handleMouseMove">Move mouse here</div>
</template>
<script>
export default {
methods: {
handleMouseMove(event) {
console.log('Mouse moved:', event.clientX, event.clientY);
}
}
}
</script>
- Issue: Continuous firing of mousemove events may impact performance, especially with complex event handling or frequent updates.
- Solution: Optimize mousemove event listeners and use debouncing or throttling techniques to improve performance.
@dblclick
- Use: Fires when an element is double-clicked, useful for implementing actions that require a rapid user interaction.
- Example:
<template>
<div @dblclick="handleDoubleClick">Double-click me</div>
</template>
<script>
export default {
methods: {
handleDoubleClick() {
console.log('Double-clicked!');
}
}
}
</script>
- Issue: Incorrect handling of dblclick events may result in unexpected behavior or conflicts with other interactions.
- Solution: Ensure proper event handling and consider user expectations for consistent behavior.
Conclusion
The power of interactivity is in your hands. Armed with the knowledge of essential events, you’re ready to captivate users with seamless experiences that respond to their every move. Whether it’s handling clicks, tracking mouse movements, or managing keyboard shortcuts, Vue.js events empower you to create applications that feel intuitive, responsive, and downright delightful. So go ahead, experiment, explore, and let the magic of Vue.js events elevate your next project to new heights. Explore Vue.js 2 and Vue.js 3 .
Remember: The Vue ecosystem is vast and ever-evolving. Stay curious, explore the official Vue documentation https://vuejs.org/guide/introduction.html , experiment with different libraries and tools, and actively engage with the vibrant Vue community. With dedication and practice, you’ll be building remarkable Vue applications in no time!
Stay tuned every Tuesday for fresh insights into frontend topics, because keeping up with the latest in web development has never been more exciting!